날짜 데이터로 검색할 때 오늘, 어제, 3일 전, 한 달 전, 당월의 날짜를 버튼으로 입력하고 입력한 날짜 클릭 시 jQuery Datepicker를 사용해서 날짜를 달력 형태로 수정할 수 있는 폼을 구현하는 코드에 대하여 설명하겠다.
1. jQuery 및 jQuery UI 스크립트 태그 추가
파일 최상단 또는 Head 파일에 다음과 같이 작성하여 jQuery와 jQuery UI를 사용하기 위해 추가한다.
<script src="https://code.jquery.com/jquery-3.6.1.js" integrity="sha256-3zlB5s2uwoUzrXK3BT7AX3FyvojsraNFxCc2vC/7pNI=" crossorigin="anonymous"></script> <script src="https://code.jquery.com/ui/1.13.1/jquery-ui.min.js" integrity="sha256-eTyxS0rkjpLEo16uXTS0uVCS4815lc40K2iVpWDvdSY=" crossorigin="anonymous"></script> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jqueryui/1.12.1/jquery-ui.min.css" integrity="sha512-aOG0c6nPNzGk+5zjwyJaoRUgCdOrfSDhmMID2u4+OIslr0GjpLKo7Xm0Ao3xmpM4T8AmIouRkqwj1nrdVsLKEQ==" crossorigin="anonymous" referrerpolicy="no-referrer" />
2. 날짜 입력 버튼 및 폼 작성
날짜 입력 폼의 기본값인 현재 날짜는 PHP 내장 함수 date를 사용해서 Y-m-d(년-월-일) 형식으로 표시한다.
<table> <tr> <td> <button type="button" onclick="get0day()">오늘</button> <button type="button" onclick="get1Day()">어제</button> <button type="button" onclick="get3Day()">3일전</button> <button type="button" onclick="get1Month()">한달전</button> <button type="button" onclick="get0Month()">당월</button> </td> </tr> <tr> <td> <label for="cal01" class="datepicker"> <input type="text" name="search_sdate" id="cal01" value="<?=date("Y-m-d");?>"> </label> ~ <label for="cal02" class="datepicker"> <input type="text" name="search_edate" id="cal02" value="<?=date("Y-m-d");?>"> </label> </td> </tr> </table> <script type="text/javascript" src="동작 구현 파일 경로"></script>
3. 동작 구현 파일 작성
날짜 입력 버튼과 Datepicker의 동작을 구현할 JavaScript 파일을 작성한다.
function get0day(){ // 오늘 var nowDate = new Date(); var nowYear = nowDate.getFullYear(); var nowMonth = nowDate.getMonth() + 1; var nowDay = nowDate.getDate(); if(nowMonth < 10){ nowMonth = "0" + nowMonth; } if(nowDay < 10) { nowDay = "0" + nowDay; } var todayDate = nowYear + "-" + nowMonth + "-" + nowDay; $("#cal01").val(todayDate); $("#cal02").val(todayDate); } function get1Day(){ //어제 var nowDate = new Date(); var yesterDate = nowDate.getTime() - (1 * 24 * 60 * 60 * 1000); nowDate.setTime(yesterDate); var yesterYear = nowDate.getFullYear(); var yesterMonth = nowDate.getMonth() + 1; var yesterDay = nowDate.getDate(); if(yesterMonth < 10){ yesterMonth = "0" + yesterMonth; } if(yesterDay < 10) { yesterDay = "0" + yesterDay; } var resultDate = yesterYear + "-" + yesterMonth + "-" + yesterDay; $("#cal01").val(resultDate); $("#cal02").val(resultDate); } function get3Day(){ // 3일전 //시작일자 var nowDate = new Date(); var weekDate = nowDate.getTime() - (3 * 24 * 60 * 60 * 1000); nowDate.setTime(weekDate); var weekYear = nowDate.getFullYear(); var weekMonth = nowDate.getMonth() + 1; var weekDay = nowDate.getDate(); if(weekMonth < 10){ weekMonth = "0" + weekMonth; } if(weekDay < 10) { weekDay = "0" + weekDay; } var resultDate = weekYear + "-" + weekMonth + "-" + weekDay; $("#cal01").val(resultDate); //종료일자 var nowDate = new Date(); var weekDate = nowDate.getTime() - (1 * 24 * 60 * 60 * 1000); nowDate.setTime(weekDate); var weekYear = nowDate.getFullYear(); var weekMonth = nowDate.getMonth() + 1; var weekDay = nowDate.getDate(); if(weekMonth < 10){ weekMonth = "0" + weekMonth; } if(weekDay < 10) { weekDay = "0" + weekDay; } var resultDate = weekYear + "-" + weekMonth + "-" + weekDay; $("#cal02").val(resultDate); } function get1Month(){ // 한달전 //시작일자 var nowDate = new Date(); var monthDate = nowDate.getTime() - (30 * 24 * 60 * 60 * 1000); nowDate.setTime(monthDate); var monthYear = nowDate.getFullYear(); var monthMonth = nowDate.getMonth() + 1; var monthDay = nowDate.getDate(); if(monthMonth < 10){ monthMonth = "0" + monthMonth; } if(monthDay < 10) { monthDay = "0" + monthDay; } var resultDate = monthYear + "-" + monthMonth + "-" + monthDay; $("#cal01").val(resultDate); //종료일자 var nowDate = new Date(); var yesterDate = nowDate.getTime() - (1 * 24 * 60 * 60 * 1000); nowDate.setTime(yesterDate); var yesterYear = nowDate.getFullYear(); var yesterMonth = nowDate.getMonth() + 1; var yesterDay = nowDate.getDate(); if(yesterMonth < 10){ yesterMonth = "0" + yesterMonth; } if(yesterDay < 10) { yesterDay = "0" + yesterDay; } var resultDate = yesterYear + "-" + yesterMonth + "-" + yesterDay; $("#cal02").val(resultDate); } function get0Month(){ // 당월 //시작일자 var nowDate = new Date(); var weekYear = nowDate.getFullYear(); var weekMonth = nowDate.getMonth() + 1; var weekDay = new Date(nowDate.getYear(), nowDate.getMonth()); weekDay = weekDay.getDate(); if(weekMonth < 10){ weekMonth = "0" + weekMonth; } if(weekDay < 10) { weekDay = "0" + weekDay; } var resultDate = weekYear + "-" + weekMonth + "-" + weekDay; $("#cal01").val(resultDate); //종료일자 var nowDate = new Date(); var weekYear = nowDate.getFullYear(); var weekMonth = nowDate.getMonth() + 1; var weekDay = (new Date(weekYear, weekMonth, 0)).getDate(); if(weekMonth < 10){ weekMonth = "0" + weekMonth; } var resultDate = weekYear + "-" + weekMonth + "-" + weekDay; $("#cal02").val(resultDate); } $(function(){ $(".datepicker > input").datepicker({ closeText: "닫기", prevText: "이전달", nextText: "다음달", currentText: "오늘", monthNames: ["1월", "2월", "3월", "4월", "5월", "6월","7월", "8월", "9월", "10월", "11월", "12월"], monthNamesShort: ["1월", "2월", "3월", "4월", "5월", "6월", "7월", "8월", "9월", "10월", "11월", "12월"], dayNames: ["일요일", "월요일", "화요일", "수요일", "목요일", "금요일", "토요일"], dayNamesShort: ["일", "월", "화", "수", "목", "금", "토"], dayNamesMin: ["일", "월", "화", "수", "목", "금", "토"], weekHeader: "주", dateFormat: "yy-mm-dd", firstDay: 0, isRTL: false, showMonthAfterYear: true, yearSuffix: "년" }); });
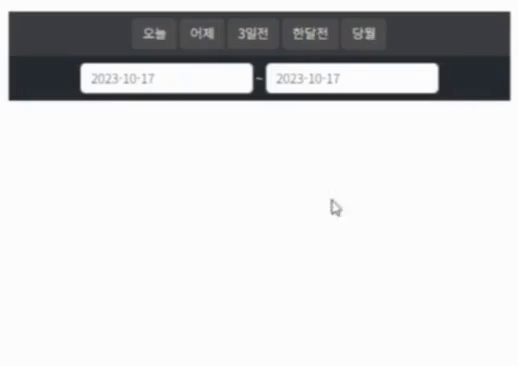
날짜 입력 버튼과 Datepicker가 잘 작동하는지 확인한다.
Datepicker 기본 스킨은 촌스러워서 여기를 참고하여 깔끔한 디자인으로 수정했다.
⦁ date(
string $format
, ?int $timestamp = null);
$timestamp가 null일 경우에는 현재 시각을 주어진 포맷 문자열에 따라 문자열로 반환한다.
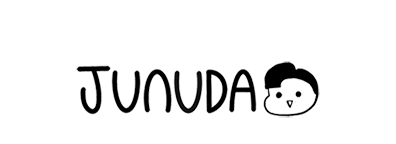